
Scenario: Say you want to intercept a response from any WordPress API call before it gets returned to the user? For example, you want to block /users/ route or append additional content to a /posts/ response. Simply call the rest_pre_echo_response filter and you can modify all responses!
Demo
In our demo we are going to block all requests to the /users/ endpoint for those who aren’t logged in
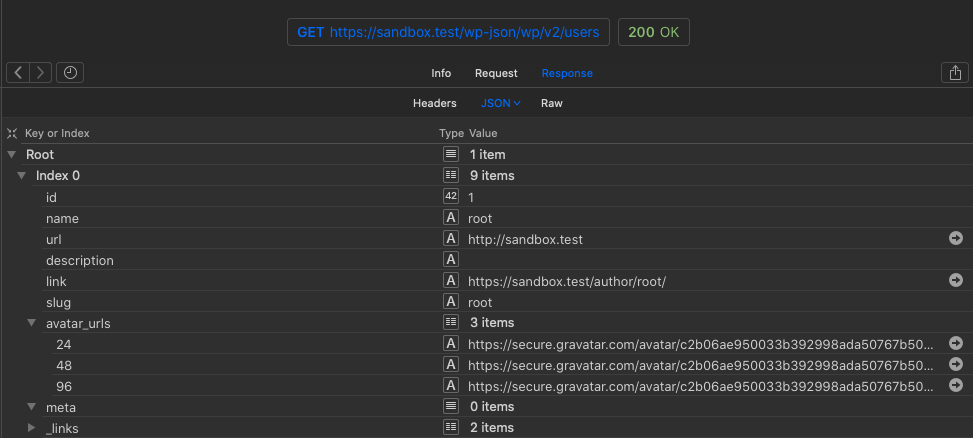
All we have to do is create a callback function for the rest_pre_echo_response filter. And from there we can check $request->get_route() to get the route that is being requested. In this example we are simply returning our own output, however you can make additional query calls and simply modify the $results array if you want the original request to continue just modified with your changes with an array_merge.
<?php
/**
* Plugin Name: Disable Users API
* Description: Disables public access to the WordPress /wp/v2/users/* api routes.
*
* @author Scott Anderson <[email protected]>
* @package ThriftyDeveloper\MU_Plugin\SandBox\Diable_Users_API
* @since NEXT
*/
namespace ThriftyDeveloper\MU_Plugin\SandBox;
/**
* Disables public access to the WordPress /wp/v2/users/* api routes.
*
* @author Scott Anderson <[email protected]>
* @since NEXT
*
* @param array $result Results data from API request.
* @param \WP_REST_Server $server Server instance.
* @param \WP_Rest_Request $request Incoming Request.
*
* @return array
*/
function lockdown_users_api( array $result, \WP_REST_Server $server, \WP_Rest_Request $request ) : array {
if( strpos( $request->get_route(), '/v2/users' ) !== false && ! is_user_logged_in() ) {
return [
'succes' => false,
'message' => __( 'You must be logged in to access /v2/users/* routes.', 'td-lockdown-users-api' ),
];
}
return $result;
}
add_filter( 'rest_pre_echo_response', 'ThriftyDeveloper\MU_Plugin\SandBox\lockdown_users_api', 10, 3 );
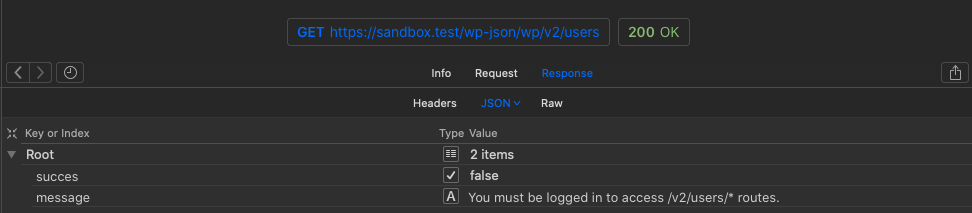
Das It
